Package Exports
- ts-toolbelt
This package does not declare an exports field, so the exports above have been automatically detected and optimized by JSPM instead. If any package subpath is missing, it is recommended to post an issue to the original package (ts-toolbelt) to support the "exports" field. If that is not possible, create a JSPM override to customize the exports field for this package.
Readme
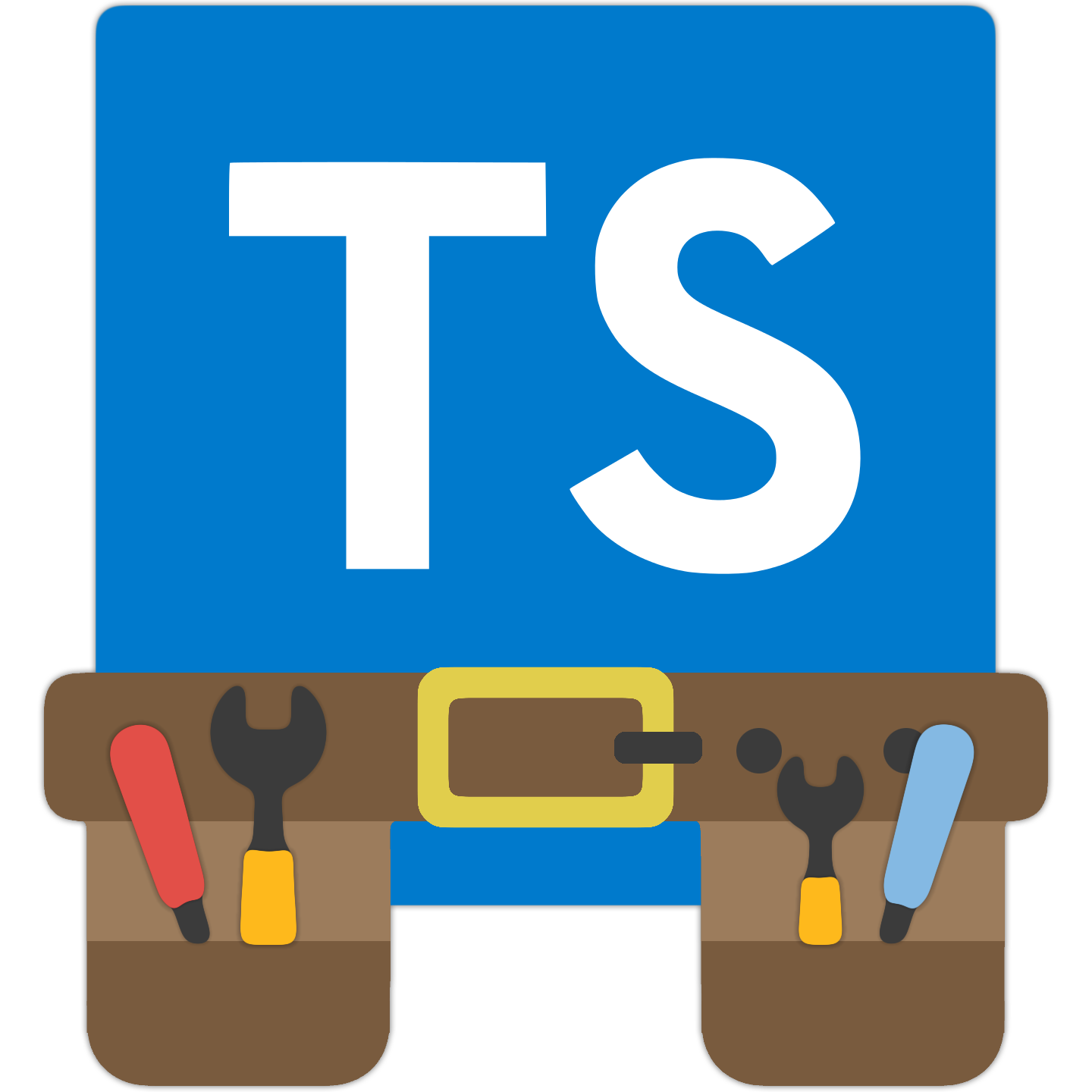
Installation
npm install ts-toolbelt --save
yarn add ts-toolbelt
import * as T from 'ts-toolbelt'
// Do basic number operations
type test0 = T.N.Minus<'5', '20'> // -15
type O = {
a: {
b: {
c: 'hello'
d: 'goodbye'
}
}
}
// Deeply change a type
type test1 = T.O.P.Update<O, ['a', 'b', 'c' | 'd'], [1, 2, 3]>
// Or just merge them
type test2 = T.O.Merge<O, {x: 'hello'}>
And so much more... But before you start reading the docs:
The library is organized around TypeScript concepts:
- T.A: Any types
- T.B: Boolean types
- T.C: Class types
- T.F: Funtion types
- T.I: Iteration types
- T.N: Number types
- T.O: Object types
- T.S: String types
- T.T: Tuple types
- T.U: Union types
type test3 = T.A.Is<'hello', string> // true
type test4 = T.T.Concat<[1, 2], [3, 4]> // [1, 2, 3, 4]