Package Exports
- pcall.js
Readme
Pcall.js
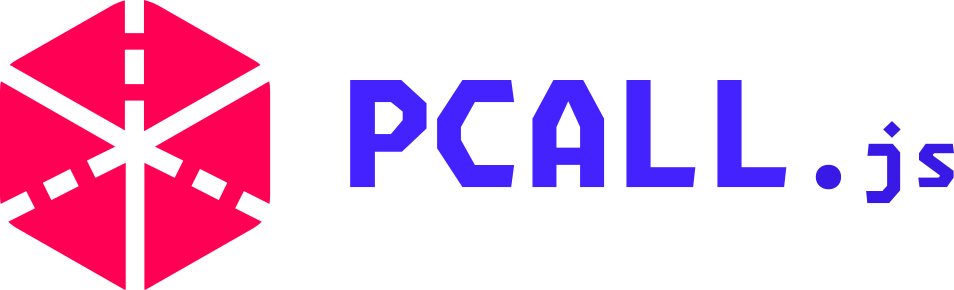
Errors as Values
zero-dependency
βΆββ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆββ΄- π Delegate Promise Resolutions
- 𧬠Lifecycle Callback Options
- π Concise and Convenient Signature
- π¦ Zero-Dependency
- π Avoid try/catch ~~HELL~ πΉ
- π Better Visibility and Control
- π Works in Node.js (ESM/CJS) and all modern browsers
- γ½οΈ Minimal Obsessive Disorder
simple

options

custom

create new safe utilities with shared side-effect behavior
Inspiration
Lua approach to error handling is simple yet powerful. ^Lua:5.4 ^Lua:8.4, ^Lua:8.5
πΉ pcall.js
is heavily inspired by Lua pcall
with superpowers π¦!
SYNOPSIS
pcall({f}, {arg1}, {...})
pcall()
Calls function {f}
with the given arguments in protected mode.
This means that any error inside {f}
is not propagated;
Instead, pcall
catches the error and returns a tuple.
Its first element is the status code (a boolean),
which is true if the call succeeds without errors.
And all results from the call, on second element; [true, {res}]
In case of any error, pcall returns false plus the error message; [false, {err}]
Usage
# install
npm install pcall.js
// ESM
import Pcall from 'pcall.js'
// CJS
const Pcall = require('pcall.js')
Convert
// π» BEFORE
try {
const res = await readFile('./package.json', { encoding: 'utf8' })
} catch(error) {
console.error(error, 'π₯')
}
// βββββββββββββββββ
// πΉAFTER
import Pcall from 'pcall.js'
const [err, user] = await Pcall(readFile, './package.json', { encoding: 'utf8' })
// πΈTHROW
err && throw new Error("XYZZY", { cause: err });
Options
import { readFile } from 'node:fs/promises'
import Pcall from 'pcall.js'
const path = './package.json'
const opts = { encoding: 'utf8' }
const pcall = new Pcall({
onSuccess: (args, res) => { /*Β·πΉΒ·*/ },
onFailure: (args, err) => { /*Β·πΉΒ·*/ },
transformOnSuccess: (args, res) => { /*Β·πΉΒ·*/ },
transformOnFailure: (args, err) => { /*Β·πΉΒ·*/ },
cleanup: (err, res, args) => { /*Β·πΉΒ·*/ },
happy: false, // true will only return result
trace: true,
})
const [err, res] = await pcall(readFile, path, opts)
console.log('err', '::', err)
console.log('res', '::', res)
Example
import { readFile } from 'node:fs/promises'
import Pcall from 'pcall.js'
const path = './test/pcall.test.json'
const opts = { encoding: 'utf8' }
const pcall = new Pcall({
onSuccess: (args, res) => log('@:SUCCESS', { args, res }),
onFailure: (args, err) => log('@:FAILURE', { args, err }),
transformOnSuccess: (args, res) => JSON.parse(res),
transformOnFailure: (args, cause) => new Error('BAD', { cause }),
cleanup: (err, res, args) => log('@CLEANUP', { err, res, args }),
trace: true,
})
const [err, res] = await pcall(readFile, path, opts)
console.error(':Pcall:[ERR]:<<', err, '>>')
console.debug(':Pcall:[RES]:<<', res, '>>')
Example Custom
import { readFile } from 'node:fs/promises'
const path = './test/pcall.test.json'
const opts = { encoding: 'utf8' }
const pread = new Pcall({
transformOnSuccess: (args, res) => JSON.parse(res),
fn: readFile,
})
const [err, res] = await pread(path, opts)
console.error(':Pcall:[ERR]:<<', err, '>>')
console.debug(':Pcall:[RES]:<<', res, '>>')
Example Happy
import { readFile } from 'node:fs/promises'
const path = './test/pcall.test.json'
const opts = { encoding: 'utf8' }
const pcall = new Pcall({
transformOnSuccess: (args, res) => JSON.parse(res),
happy: true,
})
const res = await pcall(readFile, path, opts)
console.log(res.hogo) // fuga
π‘ Check [test/pcall.canary.js]
Development
# run test playground in watch mode
npm run dev
# build production
npm run build
# build stub
npm run build:stub
TODO
- π Lifecycle Hooks
- [.] π Serializer
- [.] 𧬠Parser
- [.] π JSDoc
- [.] π§ ESLint
- [o] π Docs
- [o] β οΈ Tests
- [.] π‘ Examples