Package Exports
- pcall.js
Readme
PCALL.js
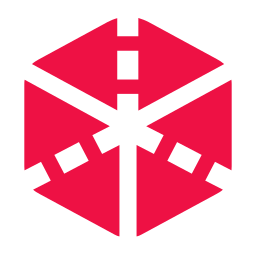
Errors as Values
unwrap/expand to array-like safe tuple results
βΆββ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆβ΄βΆββ΄π§¬ Lifecycle Hooks
π¦ Zero Dependency
π Concise Signature
π Group Side Effects
π try/catch ~~HELL~ πΉ
π Better Visibility and Control
π Works in ESM & CJS
γ½οΈ Minimal Obsessive Disorder
Inspiration
pcall.js
is heavily inspired by
πΉ Lua pcall
status, res
πΉ Elixir Error Monad {:error, reason} | {:ok, value}
πΉ Rust Result<T, E>
πΉ Go []error
with superpowers π¦!
In Lua Errors are detected and explained in terms of Lua. ^Lua:5.4 ^Lua:8.4, ^Lua:8.5
You can contrast that with C, where the behavior of many wrong programs can only be explained in terms of the underling hardware and error positions are given as a program counter
Activities start from a call by the application, usually asking to run a chunk.
If there is any error, this call returns an error code and the application can take appropriate actions
SYNOPSIS
pcall({f}, {arg1}, {...})
pcall()
Calls function {f}
with the given arguments in protected mode.
This means that any error inside {f}
is not propagated;
Instead, pcall
catches the error and returns a tuple.
Its first element is the error code status code (a boolean),
Which is false
if the call succeeds without errors.
And all results from the call, on second element; [false, {res}]
In case of any error,
Pcall returns true
plus the error message; [true, {err}]
Usage
# install
npm install pcall.js
// ESM
import Pcall from 'pcall.js'
// CJS
const Pcall = require('pcall.js')
Convert
import { readFile } from 'node:fs/promises'
// π» BEFORE
try {
const res = await readFile('./package.json', { encoding: 'utf8' })
} catch(error) {
console.error(error, 'π₯')
}
// βββββββββββββββββ
// πΉAFTER
import Pcall from 'pcall.js'
const [err, res] = await Pcall(readFile, './package.json', { encoding: 'utf8' })
// πΈTHROW
err && throw new Error("XYZZY", { cause: err });
// βββββββββββββββββ
// πΈ NO @ITERATOR
import Pcall from 'pcall.js'
const pcall = new Pcall({ noError: true })
const res = await pcall(readFile, './package.json', { encoding: 'utf8' })
// βββββββββββββββββ
// πΈ MOCK
const readJson = new Pcall({
fn: readFile,
noError: true,
args: [{ encoding: 'utf8' }],
transformOnSuccess: (args, res) => JSON.parse(res),
transformOnFailure: (args, { name, message }) => ({ name, message }),
})
const path = 'test/sample-good.json'
const res = await readJson(path)
log(res.hogo) // fuga
Options
import { readFile } from 'node:fs/promises'
import Pcall from 'pcall.js'
const pcall = new Pcall({
onSuccess: console.log,
onFailure: console.error,
onFinally: (args) => { /* π£ π£ π₯ */ },
transformOnSuccess: (args, res) => res,
transformOnFailure: (args, err) => err,
noError: false,
noTrace: false,
})
const path = './package.json'
const opts = { encoding: 'utf8' }
const [err, res] = await pcall(readFile, path, opts)
π‘ Check test/ files for more examples
Development
# run test playground in watch mode
npm run dev
# build production
npm run build
# build stub
npm run build:stub
TODO
- π Lifecycle Hooks
- [.] π Serializer
- [.] 𧬠Parser
- [.] π JSDoc
- [.] π§ ESLint
- [o] π Docs
- [o] β οΈ Tests
- [.] π‘ Examples