Package Exports
- react-select-event
This package does not declare an exports field, so the exports above have been automatically detected and optimized by JSPM instead. If any package subpath is missing, it is recommended to post an issue to the original package (react-select-event) to support the "exports" field. If that is not possible, create a JSPM override to customize the exports field for this package.
Readme
react-select-event
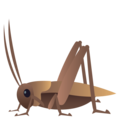
Simulate user events on react-select elements, for use with react-testing-library.
Install
npm install --save-dev react-select-event
Import react-select-event
in your unit tests:
import selectEvent from "react-select-event";
// or
const selectEvent = require("react-select-event");
Supported versions of react-select
This library is tested against all versions of react-select
starting from 2.1.0
.
API
Every helper exported by react-select-event
takes a handle on the react-select
input field as its first argument. For instance, this can be: getByLabelText("Your label name")
.
select(input: HTMLElement, optionOrOptions: string | Array<string>): Promise<void>
Select one or more values in a react-select dropdown.
const { getByTestId, getByLabelText } = render(
<form data-testid="form">
<label htmlFor="food">Food</label>
<Select options={OPTIONS} name="food" inputId="food" isMulti />
</form>
);
expect(getByTestId("form")).toHaveFormValues({ food: "" });
await selectEvent.select(getByLabelText("Food"), ["Strawberry", "Mango"]);
expect(getByTestId("form")).toHaveFormValues({ food: ["strawberry", "mango"] });
await selectEvent.select(getByLabelText("Food"), "Chocolate");
expect(getByTestId("form")).toHaveFormValues({
food: ["strawberry", "mango", "chocolate"]
});
This also works for async selects:
const { getByTestId, getByLabelText } = render(
<form data-testid="form">
<label htmlFor="food">Food</label>
<Async
options={[]}
loadOptions={fetchTheOptions}
name="food"
inputId="food"
isMulti
/>
</form>
);
expect(getByTestId("form")).toHaveFormValues({ food: "" });
// start typing to trigger the `loadOptions`
fireEvent.change(getByLabelText("Food"), { target: { value: "Choc" } });
await selectEvent.select(getByLabelText("Food"), "Chocolate");
expect(getByTestId("form")).toHaveFormValues({
food: ["chocolate"]
});
create(input: HTMLElement, option: string): void
Creates and selects a new item. Only applicable to react-select
Creatable
elements.
const { getByTestId, getByLabelText } = render(
<form data-testid="form">
<label htmlFor="food">Food</label>
<Creatable options={OPTIONS} name="food" inputId="food" />
</form>
);
expect(getByTestId("form")).toHaveFormValues({ food: "" });
await selectEvent.create(getByLabelText("Food"), "papaya");
expect(getByTestId("form")).toHaveFormValues({ food: "papaya" });
clearFirst(input: HTMLElement): void
Clears the first value in the dropdown.
const { getByTestId, getByLabelText } = render(
<form data-testid="form">
<label htmlFor="food">Food</label>
<Creatable
defaultValue={OPTIONS[0]}
options={OPTIONS}
name="food"
inputId="food"
isMulti
/>
</form>
);
expect(getByTestId("form")).toHaveFormValues({ food: "chocolate" });
selectEvent.clearFirst(getByLabelText("Food"));
await wait(() => {
expect(getByTestId("form")).toHaveFormValues({ food: "" });
});
clearAll(input: HTMLElement): void
Clears all values in the dropdown.
const { getByTestId, getByLabelText } = render(
<form data-testid="form">
<label htmlFor="food">Food</label>
<Creatable
defaultValue={[OPTIONS[0], OPTIONS[1], OPTIONS[2]]}
options={OPTIONS}
name="food"
inputId="food"
isMulti
/>
</form>
);
expect(getByTestId("form")).toHaveFormValues({
food: ["chocolate", "vanilla", "strawberry"]
});
selectEvent.clearFirst(getByLabelText("Food"));
await wait(() => {
expect(getByTestId("form")).toHaveFormValues({ food: "" });
});
Credits
All the credit goes to Daniel and his StackOverflow answer: https://stackoverflow.com/a/56085734.