Package Exports
- @datastructures-js/set
This package does not declare an exports field, so the exports above have been automatically detected and optimized by JSPM instead. If any package subpath is missing, it is recommended to post an issue to the original package (@datastructures-js/set) to support the "exports" field. If that is not possible, create a JSPM override to customize the exports field for this package.
Readme
@datastructures-js/set
extends javascript ES6 global Set class and implements new functions in it.
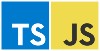
Table of Contents
Install
npm install --save @datastructures-js/set
require
const { EnhancedSet } = require('@datastructures-js/set');
import
import { EnhancedSet } from '@datastructures-js/set';
API
constructor
JS
const set1 = new EnhancedSet(['A', 'B', 'C', 'D']);
const set2 = new EnhancedSet(['C', 'D', 'E', 'F']);
TS
const set1 = new EnhancedSet<string>(['A', 'B', 'C', 'D']);
const set2 = new EnhancedSet<string>(['C', 'D', 'E', 'F']);
.union(set)
applies union with another set and returns a set with all elements of the two.
params | return | runtime |
---|---|---|
set: EnhancedSet<T> | EnhancedSet<T> |
O(n+m)
n = number of elements of the current set m = number of elements of the input set |
console.log(set1.union(set2)); // EnhancedSet { 'A', 'B', 'C', 'D', 'E', 'F' }
.intersect(set)
applies intersection between the set and another set and returns the existing elements in both.
params | return | runtime |
---|---|---|
set: EnhancedSet<T> | EnhancedSet<T> |
O(n)
n = number of elements of the current set |
console.log(set1.intersect(set2)); // EnhancedSet { 'C', 'D' }
.complement(set)
finds the complement of a set from the set elements.
params | return | runtime |
---|---|---|
set: EnhancedSet<T> | EnhancedSet<T> |
O(n)
n = number of elements of the current set |
console.log(set1.complement(set2)); // EnhancedSet { 'A', 'B' }
console.log(set2.complement(set1)); // EnhancedSet { 'E', 'F' }
.isSubsetOf(set)
checks if the set is a subset of another set and returns true if all elements of the set exist in the other set.
params | return | runtime |
---|---|---|
set: EnhancedSet<T> | boolean |
O(n)
n = number of elements of current set |
console.log(set1.isSubsetOf(new Set(['A', 'B', 'C', 'D', 'E']))); // true
console.log(set1.isSubsetOf(set2)); // false
.isSupersetOf(set)
checks if the set is a superset of another set and returns true if all elements of the other set exist in the set.
params | return | runtime |
---|---|---|
set: EnhancedSet<T> | boolean |
O(n)
n = number of elements of current set |
console.log(set1.isSupersetOf(new Set(['A', 'B']))); // true
console.log(set1.isSupersetOf(set2)); // false
.product(set[, separator])
applies cartesian product between two sets. Default separator is empty string ''.
params | return | runtime |
---|---|---|
set: Set<any>
separator: string |
EnhancedSet<string> |
O(n*m)
n = number of elements of the current set m = number of elements of the input set |
console.log(set1.product(set2));
/*
EnhancedSet {
'AC',
'AD',
'AE',
'AF',
'BC',
'BD',
'BE',
'BF',
'CC',
'CD',
'CE',
'CF',
'DC',
'DD',
'DE',
'DF'
}
*/
console.log(set1.product(set2, ','));
/*
EnhancedSet {
'A,C',
'A,D',
'A,E',
'A,F',
'B,C',
'B,D',
'B,E',
'B,F',
'C,C',
'C,D',
'C,E',
'C,F',
'D,C',
'D,D',
'D,E',
'D,F'
}
*/
.power(m[, separator])
applies cartesian product on the set itself. It projects the power concept on sets and also accepts a separator with default empty string value ''.
params | return | runtime |
---|---|---|
m: number
separator: string |
EnhancedSet<string> |
O(n^m)
n = number of elements of the set m = the multiplication power number |
const x = new EnhancedSet(['A', 'B']);
const y = x.power(2);
console.log(y);
/*
EnhancedSet(4) [Set] {
'AA',
'AB',
'BA',
'BB'
}
*/
const z = y.power(2);
console.log(z);
/*
EnhancedSet(16) [Set] {
'AAAA',
'AAAB',
'AABA',
'AABB',
'ABAA',
'ABAB',
'ABBA',
'ABBB',
'BAAA',
'BAAB',
'BABA',
'BABB',
'BBAA',
'BBAB',
'BBBA',
'BBBB'
}
*/
.permutations(m[, separator])
generates m permutations from the set elements. It also accepts a separator with default empty string value ''.
params | return | runtime |
---|---|---|
m: number
separator: string |
EnhancedSet<string> |
O(n^m)
n = number of elements of the set m = number of permutations |
const x = new EnhancedSet(['A', 'B', 'C', 'D']);
const y = x.permutations(2);
console.log(y);
/*
EnhancedSet(12) [Set] {
'AB',
'AC',
'AD',
'BA',
'BC',
'BD',
'CA',
'CB',
'CD',
'DA',
'DB',
'DC'
}
*/
.equals(set)
checks if two sets are equal.
params | return | runtime |
---|---|---|
set: Set<any> | boolean |
O(n)
n = number of elements of the current set |
console.log(set1.equals(new Set(['B', 'A', 'D', 'C']))); // true
console.log(set1.equals(new EnhancedSet(['D', 'C']))); // false
.filter(cb)
filters the set based on a callback and returns the filtered set.
params | return | runtime |
---|---|---|
cb: (element: T) => boolean | EnhancedSet<T> |
O(n)
n = number of elements of the set |
console.log(set1.filter((el) => el > 'B')); // EnhancedSet { 'C', 'D' }
.toArray()
converts the set into an array.
return |
---|
T[] |
console.log(set1.toArray()); // [ 'A', 'B', 'C', 'D' ]
.clone()
clones the set.
return |
---|
EnhancedSet<T> |
console.log(set1.clone()); // EnhancedSet { 'A', 'B', 'C', 'D' }
Build
grunt build
License
The MIT License. Full License is here